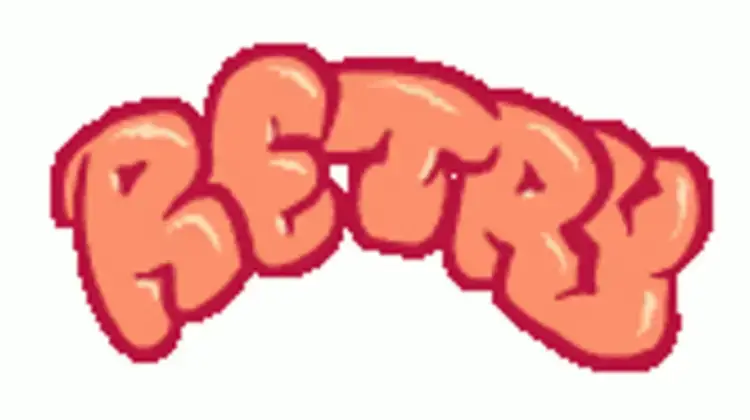
When it comes to writing code in any programming language, it’s important to handle exceptions and errors that may arise. In Python, one way to handle these exceptions is by using the “try” and “except” blocks. However, what if the error that you are encountering is a temporary one, and you want to retry the operation until it succeeds? This is where the Retry Decorator comes into play.
A Retry Decorator is a Python decorator that can be used to wrap around a function that you want to retry if an exception is raised.
It allows you to specify how many times you want to retry the operation, and the time interval between each retry.
In this blog post, we will be discussing the basics of the Retry Decorator, and how it can be used to simplify your code.
First, let’s start with the basics of the Retry Decorator.
A Python decorator is a special syntax that allows you to modify the behavior of a function.
A Retry Decorator takes advantage of this syntax to provide a way to retry a function if an exception is raised.
Here’s an example of a simple Retry Decorator:
import time def retry(max_retries, wait_time): def decorator(func): def wrapper(*args, **kwargs): retries = 0 if retries < max_retries: try: result = func(*args, **kwargs) return result except Exception as e: retries += 1 time.sleep(wait_time) else: raise Exception(f"Max retries of function {func} exceeded") return wrapper return decorator @retry(max_retries=5, wait_time=1) def example_function(): # function that may raise an exception pass
In this example, the Retry Decorator takes two arguments: max_retries and wait_time. The max_retries argument specifies the maximum number of times that the function should be retried, and the wait_time argument specifies the number of seconds to wait between each retry.
To use the Retry Decorator, you simply need to add the @retry syntax before the function definition. In this example, the example_function will be retried up to 5 times if an exception is raised, with a wait time of 1 second between each retry.
This simple example should give you an idea of how the Retry Decorator can be used to simplify your code and handle exceptions in a more efficient manner. Whether you’re working with web APIs, database connections, or any other type of operation that may fail, the Retry Decorator is a useful tool to have in your toolbox.
In conclusion, the Retry Decorator is a simple yet powerful tool that can be used to simplify your code and handle exceptions in a more efficient manner.
Hope you liked this post and learned something new today 🙂