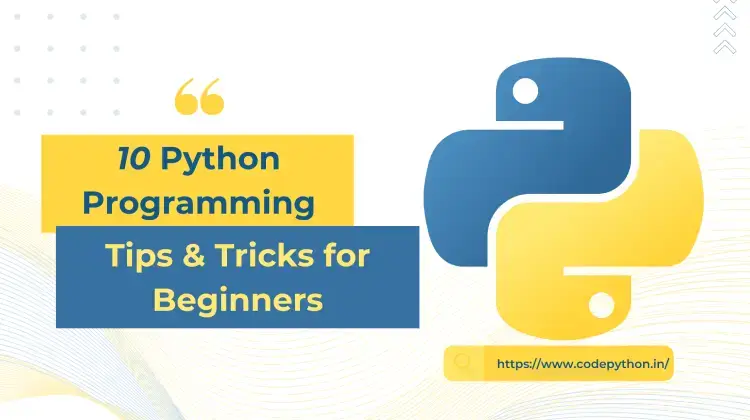
Python is a versatile and powerful programming language that is widely used in various industries. Whether you’re a beginner or an experienced programmer, there are always new tips and tricks to learn in Python.
In this blog post, we’ll take a look at 10 Python tips and tricks that are especially useful for beginners.
1. Use the built-in help() function to learn more about a specific function or module. Simply type help(function_name) in the Python interpreter to access the documentation. For example:
help(print) Help on built-in function print in module builtins: print(...) print(value, ..., sep=' ', end='\n', file=sys.stdout, flush=False) Prints the values to a stream, or to sys.stdout by default. Optional keyword arguments: file: a file-like object (stream); defaults to the current sys.stdout. sep: string inserted between values, default a space. end: string appended after the last value, default a newline. flush: whether to forcibly flush the stream.
2. Use the dir() function to see a list of all the attributes and methods of an object. This can be useful for exploring the functionality of a module or object. For example:
dir(list) ['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
3. Take advantage of list comprehensions to write more concise and readable code. List comprehensions allow you to create a new list by applying an operation to each element of an existing list. For example:
numbers = [1, 2, 3, 4, 5] squares = [x ** 2 for x in numbers] print(squares) [1, 4, 9, 16, 25]
4. Use the enumerate() function when working with lists to easily keep track of the index of an element. This function returns a tuple containing the index and the element of the list. For example:
fruits = ['apple', 'banana', 'cherry'] for i, fruit in enumerate(fruits): print(i, fruit) 0 apple 1 banana 2 cherry
5. Use the zip() function to iterate over multiple lists at the same time. `zip()
zip() returns an iterator that combines the elements of multiple lists into tuples. For example: names = ['Alice', 'Bob', 'Charlie'] ages = [25, 30, 35] for name, age in zip(names, ages): print(name, age) Alice 25 Bob 30 Charlie 35
6. Use the slice() function to extract a specific portion of a list or string. This function takes three arguments: the start index, the end index, and the step size. For example:
numbers = [1, 2, 3, 4, 5] print(numbers[1:3]) [2, 3]
7. Use the map() function to apply a function to every element of an iterable. map() returns an iterator that applies the function to each element of the iterable. For example:
numbers = [1, 2, 3, 4, 5] def square(x): return x ** 2 squares = map(square, numbers) print(list(squares)) [1, 4, 9, 16, 25]
8. Use the filter() function to filter the elements of an iterable based on a condition. filter() returns an iterator that includes only the elements that satisfy the condition. For example:
numbers = [1, 2, 3, 4, 5] def is_even(x): return x % 2 == 0 even_numbers = filter(is_even, numbers) print(list(even_numbers)) [2, 4]
9. Use the reduce() function to apply a binary function to the elements of an iterable. reduce() applies the function cumulatively to the elements, so that the first call is function(iterable[0], iterable[1]), the second call is function(function(iterable[0], iterable[1]), iterable[2]) and so on.
from functools import reduce numbers = [1, 2, 3, 4, 5] def mul(x, y): return x * y product = reduce(mul, numbers) print(product) 120
10. Finally, Practice as much as you can, try to implement the above-mentioned tricks and tips in your programs, and make sure to keep experimenting with different ways to use them in your code.
These are just a few tips and tricks to get you started with Python programming. Remember to keep experimenting and have fun with your code! With consistent practice, you will become comfortable with these tips and tricks and will be able to implement them in your code with ease.